接下來試著用 wxPython 來開發 GUI 吧!
先產生一個最陽春的視窗,
#-*- coding: utf-8 -*- #!/usr/bin/python import wx if __name__ == "__main__": app = wx.App() frame = wx.Frame(None, -1, 'wxPython Test') frame.Show() app.MainLoop()沒看錯,真的十行有找。
執行的結果,
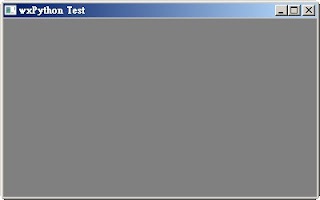
這個視窗還有很多參數可以設定,
- Move(wx.Point point)
- MoveXY(int x, int y)
- SetPosition(wx.Point point)
- SetDimensions(wx.Point point, wx.Size size)
- Centre()
- Maximize()
- Minimize()
那如果要在裡面加上其他控制項呢?
我們就要繼承這個類別,
所有的初始化就可以寫在__inti__()裡面。
#-*- coding: utf-8 -*- #!/usr/bin/python import wx class myFrame(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title, (400,300)) self.Show() if __name__ == "__main__": app = wx.App() frame = myFrame(None, -1, 'wxPython Test') app.MainLoop()執行的結果跟上面是一樣的。
2.Menu Bar
加 menu bar 的作法。
#!/usr/bin/python # -*- coding: utf-8 -*- import wx class myFrame(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title, (400,300)) menubar = wx.MenuBar() file = wx.Menu() file.Append(1, 'New', 'New a file') file.Append(1, 'Quit', 'Quit application') edit = wx.Menu() edit.Append(3, 'Copy', 'Copy') edit.Append(4, 'Paste', 'Paste') menubar.Append(file, '&File') menubar.Append(edit, '&Edit') self.SetMenuBar(menubar) self.Centre() self.Show() if __name__ == "__main__": app = wx.App() frame = myFrame(None, -1, 'wxPython Test') app.MainLoop()首先要先建立一個 MenuBar 物件,
menubar = wx.MenuBar()
接著建立 Menu
file = wx.Menu()
然後把 Menu 放到 MenuBar 裡面。
執行出來就像這樣。
在 append menu 的時候,&符號是用來建快速鍵,
當我們按 Alt 鍵,menu 的第一個字母就會出現底線,
此時我們可以接著按那個字母當作快速鍵,例如 Alt + F 或是 Alt + E。
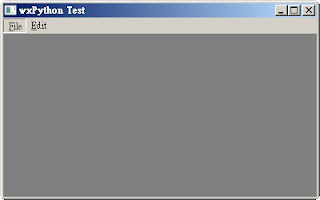
當點了某一個 menu,會產生一個 event,
當然我們必須手動把這兩個東西 bind 在一起。
#!/usr/bin/python # -*- coding: utf-8 -*- import wx class myFrame(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title, (400,300)) menubar = wx.MenuBar(wx.MB_DOCKABLE) file = wx.Menu() file.Append(1, 'New', 'New a file') file.Append(1, 'Quit', 'Quit application') edit = wx.Menu() edit.Append(3, 'Copy', 'Copy') edit.Append(4, 'Paste', 'Paste') menubar.Append(file, '&File') menubar.Append(edit, '&Edit') self.SetMenuBar(menubar) # Bind menu with an event self.Bind(wx.EVT_MENU, self.OnQuit, id=2) self.Centre() self.Show() def OnQuit(self, event): self.Close() if __name__ == "__main__": app = wx.App() frame = myFrame(None, -1, 'wxPython Test') app.MainLoop()注意 bind 的地方跟 OnQuit 這個 method 就是響應 menu 這個 event。
以上都是固定的 menu ,那如果要產生 popup menu 呢?
#-*- coding: utf-8 -*- #!/usr/bin/python import wx class MyPopupMenu(wx.Menu): def __init__(self, parent): wx.Menu.__init__(self) self.parent = parent minimize = wx.MenuItem(self, wx.NewId(), 'Minimize') self.AppendItem(minimize) self.Bind(wx.EVT_MENU, self.OnMinimize, id=minimize.GetId()) close = wx.MenuItem(self, wx.NewId(), 'Close') self.AppendItem(close) self.Bind(wx.EVT_MENU, self.OnClose, id=close.GetId()) def OnMinimize(self, event): self.parent.Iconize() def OnClose(self, event): self.parent.Close() class myFrame(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title, (400,300)) menubar = wx.MenuBar(wx.MB_DOCKABLE) file = wx.Menu() file.Append(1, 'New', 'New a file') file.Append(2, 'Quit', 'Quit application') edit = wx.Menu() edit.Append(3, 'Copy', 'Copy') edit.Append(4, 'Paste', 'Paste') menubar.Append(file, '&File') menubar.Append(edit, '&Edit') menubar.Append(MyPopupMenu(self), 'pop') self.SetMenuBar(menubar) #Bind menu with an event self.Bind(wx.EVT_MENU, self.OnQuit, id=2) #Bind right-button down event self.Bind(wx.EVT_RIGHT_DOWN, self.OnRightBtnDown) self.Centre() self.Show() def OnRightBtnDown(self, event): self.PopupMenu(MyPopupMenu(self), event.GetPosition()) def OnQuit(self, event): self.Close() if __name__ == "__main__": app = wx.App() frame = myFrame(None, -1, 'wxPython Test') app.MainLoop()有注意到嗎?
在 OnRightBtnDown 這個 method 裡面,
我們呼叫 PopupMenu ,傳進去的第一個參數是一個繼承 wx.Menu 的物件,
當然也可以是一個 wx.Menu 物件喔!
也就是說,我們也可以這樣寫:
def OnRightBtnDown(self, event): #self.PopupMenu(MyPopupMenu(self), event.GetPosition()) self.Bind(wx.EVT_MENU, self.OnTest, id=5) test = wx.Menu() test.Append(5, 'test', 'test') self.PopupMenu(test, event.GetPosition()) def OnTest(self, event): self.Close()
沒有留言:
張貼留言